Use what you learned in First NetBeans GUI to complete these exercises.
- Create an automobile information application that displays the brand of the car when its option button is selected. (Firebird is a Pontiac, Corvette is Chevrolet, Prowler is Plymouth)
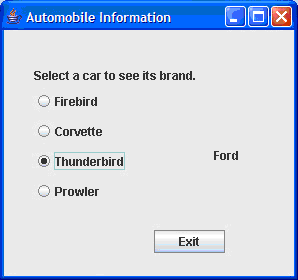
- Create an application that displays a message stating the button that was pressed.
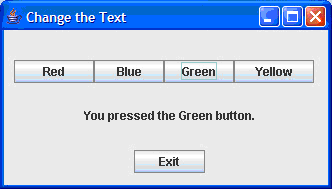
- Create an application that allows a user to enter a two-digit number and then displays the first and second digit.
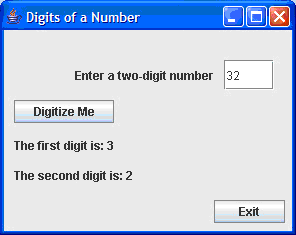
- Create an application that allows a user to enter a number less than 100 and then checks to see if the number is a one or two digit number.
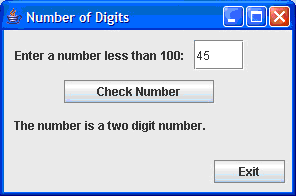
- Create an application that calculates pay based on the number of hours worked and the hourly rate. Hours worked over 40 are paid overtime at a rate of 1.5 the regular hourly rate.
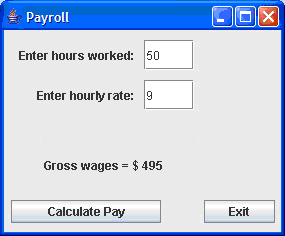
- Modify the previous application so that there is an 18% deduction from gross pay, unless the employee is exempt. The sample shown below is for an employee who is not exempt.
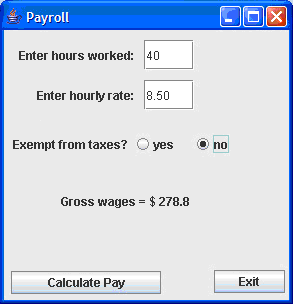
- Create an application that calculates the price per copy and the total price using the following information:
0 – 499 copies will cost $0.30 per copy
500 – 749 copies will cost $0.28 per copy
750 – 999 copies will cost $0.27 per copy
1000 copies or more will cost $0.25 per copy
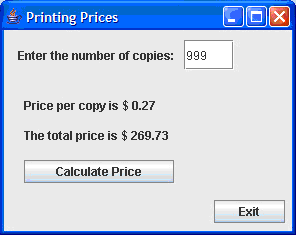
- Create an application that allows the user to enter one letter grade (uppercase or lowercase) after another. The number of students passed and the number failed should be updated by pressing the Enter Grade button after each grade is entered.
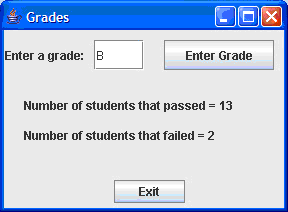
- Create an application that determines a phone bill by prompting the user for calling options (call waiting, call forwarding, and caller ID). The basic monthly charge is $25.00 and each additional calling option is $3.50.
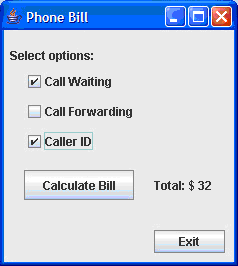
- Create an application that creates a sandwich order by prompting the user for the size of the sandwich (small or large) and the fixings (lettuce, tomato, onion, mustard, mayonnaise, cheese). A small sandwich is $2.50 and a large sandwich is $4.00. Mustard and mayonnaise are free, lettuce and onion are $0.10 each, tomato is $0.25, and cheese is $0.50. The defaults should be a small sandwich with no fixings.
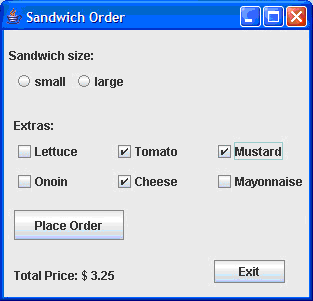
- Create an application that allows a user to enter a beginning and an ending number and then displays the total of all the numbers from the beginning number to the ending number as shown below.
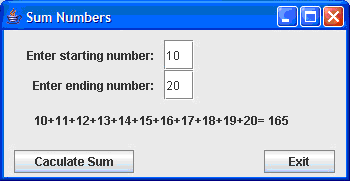
- Create an application that allows the user to enter numbers from 0 to 300 and then calculates the average each time the Calculate Average button is pressed.
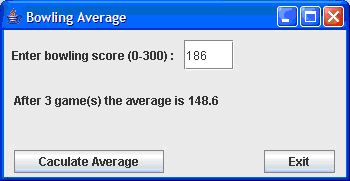
- Create an application that calculates the average of a set of numbers from 1 to a number entered by the user. For example, if the user enters 5, the average of 1,2,3,4 and 5 would be calculated.
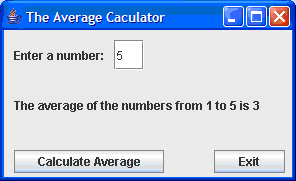
- Create an application that prompts the user to enter his or her first and last names separated by a space and then displays the initials of the name in uppercase. The following String() methods will be needed to solve the problem: trim(), toUpperCase(), indexOf(), charAt().
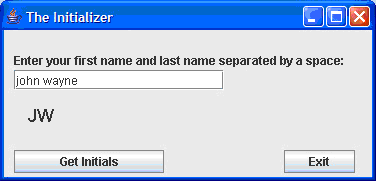
- Create an application that prompts the user to enter his or her first, middle, and last names separated by spaces and then displays the monogram as shown below. (first and middle initials in lowercase and last initial in uppercase). The following String() methods will be needed to solve the problem: trim(), toUpperCase(), toLowerCase(), indexOf(), lastIndexOf(), charAt().
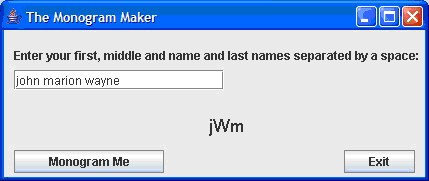
- Create an application that displays a new String in a label. The new String should take a sentence entered by the user and replace every occurrence of a substring with a new String supplied by the user. Use the String() method replaceAll() to solve the problem.
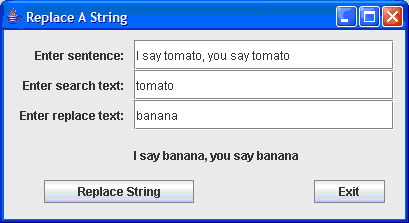
- Create an application that displays a new String in a label. The new String should take a sentence entered by the user and remover every occurrence of a substring supplied by the user. Use the String() method replaceAll() to solve the problem.
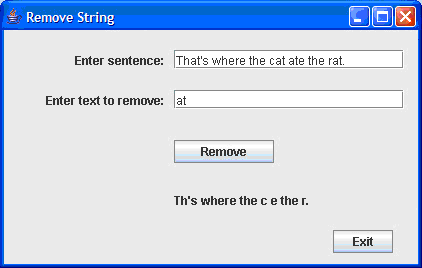
- An acronym is a word formed from the first letters of a few words, such as GUI for graphical user interface. Create an application that displays an acronym for the words entered by the user. The following String() methods will be needed to solve the problem: trim(), toUpperCase(), indexOf(), charAt().
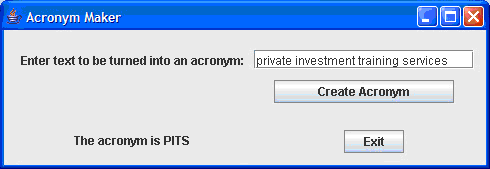
- Create an application that allows the user to enter a name and then prints it in reverse order in lowercase in a label. The following String() methods will be needed to solve the problem: trim(), toLowerCase(), charAt(), length(), concat().
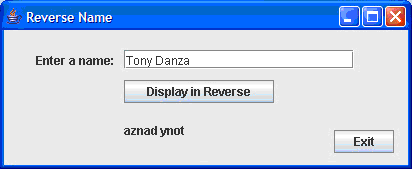
- Create an application that counts the number of vowels in a word or phrase. The following String() methods will be needed to solve the problem: trim(), charAt(), length(), concat(), compareToIgnoreCase().
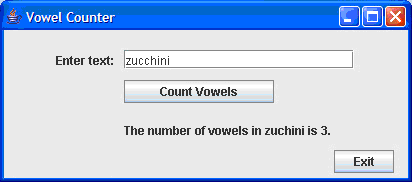
- Create an application that prompts the user for a word and then displays the Unicode base 10 number for each letter.
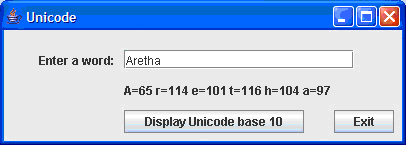
- A palindrome is a word or phrase that is spelled the same backwards and forwards, such as race car, mom, or dad. Create an application that uses a loop to determine if the word or phrase is a palindrome.
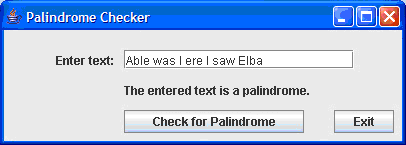
- Create an application that prompts the user to enter a student name and then displays what group a student is assigned to depending on the first letter in the student’s last name. Last names beginning with A through I are in Group 1, J through S are in Group2, and T through Z are ion Group 3.
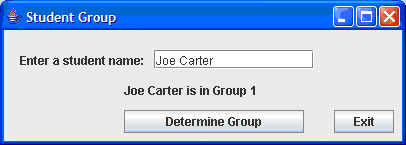
- Create an application that simulates a modified version of the game MasterMind. In this game three different colored blocks are lined up and hidden from the player. The player then tries to guess the colors and the order of the blocks. There are four colored blocks (red, green, blue, yellow) to choose from. After guessing the color of the three hidden blocks the program displays how many of the colors are correct and how many of the colors are in the right position. The players then uses the information to make more guesses until all three colors and positions are correct.
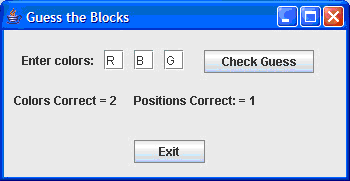
|