The Adding Game Project
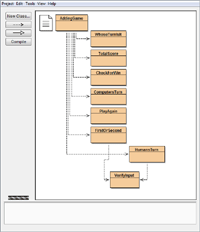
Write a program that allows the user and computer to alternately select numbers from 3 to 12. The numbers are totaled after each entry. A winner is declared when the total is greater than 100. The last one to enter a number is the winner. Have the computer ask whether the user wishes to go first or second. The computer should disallow all cheating.
Required Classes and their Methods:
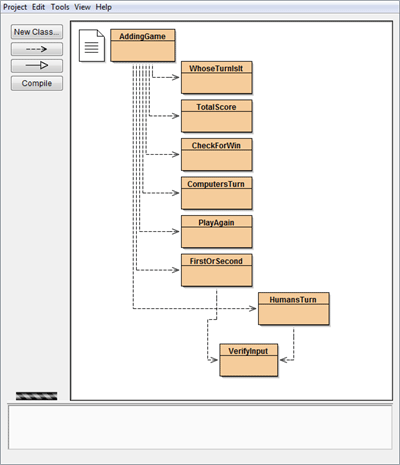
- Class VerifyInput
- Include a boolean method that returns true if the number passed in as a value parameter is from 3 to 12 and false if it is not.
- Include a boolean method that returns true if the number passed in as a value parameter is either a 1 or a 2 and false if it is not.
- Class HumansTurn
- Include an int method that returns the humans choice of a number ranging from 3 to 12. Also, the method must instantiate a VerifyInput object to check the validity of the user entry.
- Include a do while() loop that uses a VerifyInput object to validate the entry from 3 to 12. Invalid entries will cause the computer to ask the user to reenter a value from 3 to 12.
- Class ComputersTurn
- Include an int method that returns a randomly selected number from 3 to 12.
- Class PlayAgain
- Declare a boolean field variable named anotherRound.
- Include a constructor that sets the value of anotherRound to true.
- Include a void method that asks the user to play another round. The method will set the field variable anotherRound to either true or false.
- Include a boolean method that returns the value in the field variable anotherRound.
- Class CheckForWin
- Include a boolean method that accepts the current game total passed as a parameter value and returns true if the total is greater than 100 and false if it is not.
- Class TotalScore
- Declare an int field variable named total.
- Include a constructor that sets the field variable total to 0.
- Include a void method that sets the field variable total to 0. (to be used when the player wants to play again and the total needs to be reset to 0)
- Include a void method that will increase the field variable total by the amount entered by the user or the computer.
- Include an int method that returns the current value of the field variable total.
- Class WhoseTurnIsIt
- Declare a String field variable named whoseTurn.
- Create a constructor that sets the value of the field variable whoseTurn to the String "human".
- Include a String method that returns the current value of the field variable whoseTurn.
- Include a void method that sets the value of the field variable whoseTurn to a String (either human or computer) passed to it as a parameter value.
- Include a void method that changes the value of the field variable whoseTurn to “computer” if the current value is “human” or changes the value to “human” if the current value is “computer”. (this is done so that the turns will alternate between the user and the computer)
- Class FirstOrSecond
- Include an int method that asks whether the user would like to go first or second in the game. With a do while() loop the method will use a VerifyInput object to verify that the number entered is either a 1 or a 2. If an invalid response is detected the user must reenter a choice.
- Class AddingGame
- Include a main method that instantiates all the necessary objects and code for playing the adding game. Expect to use a while() loop that allows the user to continue playing as many times as desired and a do while() loop that causes any individual game to proceed until the total score is greater than 100.
(HINT: Start simple! Write the code to play a simple version of the game where the player always goes first and the game ends when the score is greater than 100. After that code is working add the code that would allow you to play again when the game is finished. Finally, add the code that would allow the user to choose whether to go first or second. Programming in small steps like this makes the work easier.)
Sample output:
Would you like to go first or second? (Enter 1 or 2): 1
Enter your choice (3-12): 10
The total is now 10
I choose 3
The total is now 13
Enter your choice (3-12): 10
The total is now 23
I choose 8
The total is now 31
…
The total is now 92.
Enter your choice (3-12): 10
The total is now 102
You have won!!!
Would you like to play another round? (Y/N): N
Goodbye.
|