Matching Game
In this exercise you will write a program that creates a matching game. During the play of the game the text of each button is hidden until the user clicks on a button. If the button is the first or second button clicked during that turn then the text becomes visible. If the text of the buttons match then the text remains visible for the rest of the game. If the text of the buttons does not match then the text of both buttons will be hidden when the next button is clicked.
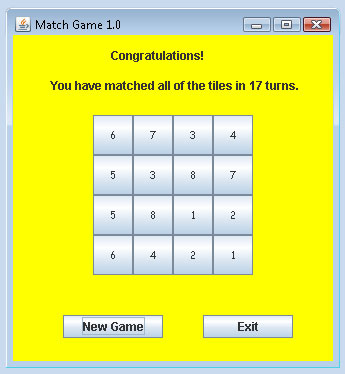
Notice: Unzip and Launch the MatchGame file to see a finished version of this project.
You will need to:
- Add 18 buttons. 1 through 16 for the grid, 1 for the New Game button and 1 for the Exit button.
- Add 2 labels. One for the turns display and one for the congratulations display.
- Use a button array to store the sixteen buttons.
- Use a String array to store the text for each of the 16 game buttons.
You must account for the following situations:
- If the user clicks on the same button more than once it should only accept the first click as legitimate. The other clicks should be ignored.
- If the user clicks on a button that already has been matched it should be ignored.
- The game should be ready to play when it opens.
Hints:
- Create a String array that has 17 indexes (0-16) so that the numbers 1-8 can twice be randomly stored as text (use Integer.toString() to convert integers to Strings).
As is customary in this class do not store anything in the 0 index. Notice in the visual representation of the puzzle_text array shown below how the stored numbers match the numbers on the buttons in the image of the game.
- Keep track of the number of buttons clicks. It is important to know if it is the first, second or third click.
- Create a void StartNewGame() method that is called from the New Game button and from the constructor.
- Create a void checkForMatch(int n) method that is called each time a game button is pressed. The method will use an integer parameter variable to accept the number of the button that was pressed.
|