In this exercise you will write a program that reproduces the classic slide puzzle.
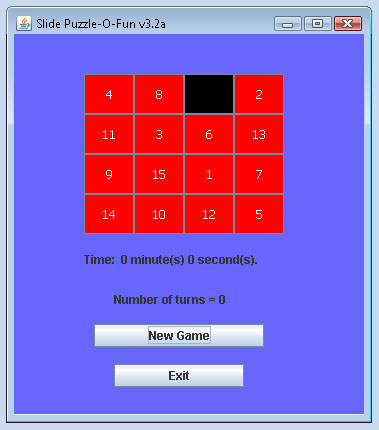
You will need to:
- Add 18 buttons. Create the first 16 buttons for the puzzle tiles, then 1 for a New Game button and 1 for an Exit button.
- Add 2 labels. One for the turns display and one for the time display.
- Use a button array to store the sixteen buttons.
- The background color of the buttons must change so that the blank button has a different background color.
- The new game button will scramble the tiles. The scrambling is not simply a random placement of the numbers. Doing that leads to puzzles that are not able to be solved.
- The numbers of turns taken to solve the puzzle must be displayed. The turns taken should be updated each time a move is made.
- The time taken to solve the puzzle must be displayed. The timer should start as soon as the first move is made. The time should be continuously displayed while the game is in progress. The timer should stop as soon as the puzzled is solved.
- The game should not start until the New Game button is pressed. In other words you should not be able to move any tiles before pressing the New Game button.
- Pressing a button that cannot be moved should not be counted as a turn
Steps:
- Unzip and Launch the SlidePuzzle file to see a finished version of this project.
- Add the sixteen buttons to the canvas. Keep them in order from jButton1 through jButton16 where the first row is jButton1 through jButton 4, the second row is jButton5 through jButton8, etc.
- Declare a field variable tile that is a JButton array to hold the sixteen buttons.
- Change the button properties to:
- background - choose a color
- font - choose a font and size
- foreground - choose a foreground color
- text - use the numbers 1 through 15 for the first 15 buttons. Button 16 the text should be deleted
- border - choose a border style if desired (BevelBorder works well)
- borderPainted - selected
- contentAreaFilled - selected
- focusPainted - deselect
- focusable - deselect
- opaque - selected
- rolloverEnabled - deselect
- Write the code so that the buttons when clicked switch colors and labels only if there is a blank space to move into. For example in the diagram below only the number 12 and number 15 tile can switch with the number 16 tile.
- use tile[12].setBackground(new java.awt.Color(255,0,0)); to set the background color of a button to red. (0,0,0) would be used for the color black.
- use tile[16].setText(tile[12].getText()); to set the text of jButton16 with what you get from the text of jButton12
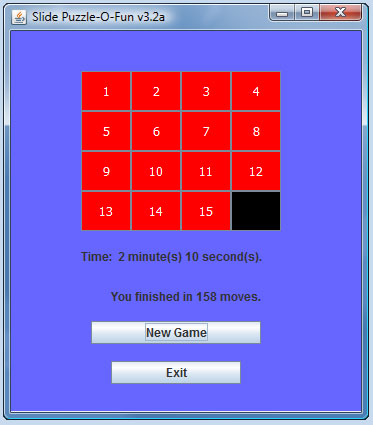
- Write the code for the New Game button.The goal is to scramble the tiles by making the computer take 1000 random moves. To simulate this you will create an array and then move the values in the array both randomly and legitimately. Simply moving the values randomly can result in puzzles that are not able to be solved.
- create an int array named scramble[] with space to hold 17 values (we will use indexes 1 through 16)
- place the value 1 in scramble[1] and the value 2 in scramble[2]. Repeat this pattern for scramble[3] through scramble[15]
- place the value 0 in scramble[16] to represent the blank tile
- create a loop that repeats for 1000 iterations:
- check for the position of the 0 value in scramble[] and then
- check to see which tiles can move into the blank spot. for example if scramble[6]==0 then there are four possible moves. either 2,5,7 or 10 can be moved into that place. choose a random number from 1 to 4 and then move the value in scramble[2,5,7 or 10] into scramble[6] and place the value 0 into scramble[2,5,7 or 10]
- after the scramble array values are set take the value in scramble[1] and make it the text for tile[1] and so forth through tile[15]
- Add a field variable that can keep track of the number of turns. The number of turns taken should be updated and displayed each time a tile swaps places with another tile.When the puzzle is finished it should display a message stating how many turns were taken.
- Write the necessary code so that the time taken to solve the puzzle can be calculated and displayed. The timer should start when the first tiles are swapped. The timer should end when the last tile is moved into the correct position
- add this code to the very top of the existing code:
import java.awt.event.*; // for ActionListener and ActionEvent to capture timer event
import javax.swing.Timer; //for Timer Class
- add this code where the field variables are located:
Timer t; //declare the Timer object
- add this code to where the field variables are located:
int w,minutes,seconds;
- add this code in the constructor just after initComponents();
//begin timer code
//this code also assumes there is a label named lblTime
t = new Timer(1000, new ActionListener(){
public void actionPerformed(ActionEvent e){
w++;
minutes=w/60;
seconds=w%60;
lblTime.setText("Time: "+minutes+" minute(s) "+seconds+" second(s).");}}); // end of timer code
- To start the timer use t.start(); and to stop the timer use t.stop();
- The field variable w needs to be reset to 0 in order to reset the timer after each game.
- Make sure that the New Game button resets the turns and time back to 0.
|
|
|