In the real world, you'll often find many individual objects all of the same kind. There may be thousands of other bicycles in existence, all of the same make and model. Each bicycle was built from the same set of blueprints and therefore contains the same components. In object-oriented terms, we say that your bicycle is an instance of the class of objects known as bicycles. A class is the blueprint from which individual objects are created.
Let's see what it would look like to make a Java program using a bicycle as an object. The following Bicycle Class is one possible implementation of a bicycle:
class Bicycle
{
// this is where field (or also called state) variables are declared.
// they can be accessed by any of the methods (global access)
int cadence; // this is a field variable
int speed; // this is a field variable
int gear; // this is a field variable
// this is a constructor for the Bicycle class
// the name of the constructor is always the same as the class name
// it is used to set the initial field variable values
Bicycle()
{
cadence = 0;// the cadence will be 0 when the Bicycle object is created
speed = 0;// the speed will be 0 when the Bicycle object is created
gear = 0;// the gear will be 0 when the Bicycle object is created
}
// this is an overloaded constructor
// it has value parameters so that a bicycle object can be created ...
// ... using values sent to it from another object
Bicycle(int theCadence, int theSpeed, int theGear)
{
cadence = theCadence;// place the value stored in theCadence into the field variable cadence
speed = theSpeed;// place the value stored in theSpeed into the field variable speed
gear = theGear;// place the value stored in theGear into the field variable gear
}
// this is a method named changeCadence()
// this method waits for an int value to be sent to it. the value will be placed in the int variable newValue
// the method will set the field variable named cadence to the value stored in newValue
// newValue is called a parameter value or variable.
// newValue is only valid in this method (local access only, not global like field variables)
void changeCadence(int newValue) // this is the name (signature) of the method
{
cadence = newValue;// place the value in newValue into cadence
}
// this is a method named changeGear()
// this method waits for an int value to be sent to it. this value is placed in the variable named newValue
// this method will set the value of the field variable gear to the value stored in the parameter
// variable named newVariable
void changeGear(int newValue)
{
gear = newValue;// place the value in newValue into gear
}
// this is a method named speedUp()
// this method waits for an int value to be sent to it. this value is placed in the variable named increment
// this method will increase the value of the field variable speed by the value stored in the parameter variable
// named increment
void speedUp(int increment)
{
speed += increment;// increase the value in speed by the value in increment
}
// this is a method named applyBrakes()
// this method waits for an int value to be sent to it. this value is placed in the parameter variable
// named decrement
// this method will decrease the value of the field variable speed by the value stored in the parameter
// variable named decrement
void applyBrakes(int decrement)
{
speed -= decrement;// decrease the value in speed by the value in decrement
}
// this is a method named printStates()
// this method does not wait for any values to be sent to it. there is not a parameter variable.
// this method will print the values of the field (or also called state) variables
void printStates()
{
System.out.println("cadence:"+cadence+" speed:"+speed+" gear:"+gear);
}
}
The syntax of the Java programming language will look new to you, but the design of this class is based on the previous discussion of bicycle objects. The fields cadence, speed, and gear represent the object's state, and the methods changeCadence, changeGear, speedUp, etc. define its interaction with the outside world.
You may have noticed that the Bicycle Class does not contain a main method. That's because it's not a complete application; it's just the blueprint for bicycles that might be used in an application. The responsibility of creating and using new Bicycle objects belongs to some other class in your application.
Here's a BicycleDemo Class that creates two separate Bicycle objects and invokes (or, calls) their methods:
/**
* this class will create (instantiate) three instances of Bicycle objects
* it only has one method, the main method
* it does not have a constructor
*/
class BicycleDemo {
static Bicycle bike1 = new Bicycle();// create an instance of the Bicycle class named bike1
static Bicycle bike2 = new Bicycle();// create an instance of the Bicycle class named bike2
// create an instance of the Bicycle class named bike3. use the overloaded constructor.
// send along the value 76 and put it into theCadence
// send along the value 28 and put it into theSpeed
// send along the value 3 and put it into theGear
static Bicycle bike3 = new Bicycle(76,28,3);
// this is the main class for the application
// it uses the other classes in the project to create the objects needed
// objects are manipulated by calling their methods and sending parameter values when needed
public static void main(String[] args)
{
// Invoke methods on the objects bike1 and bike2
System.out.print("Bike 1 starting values are -> ");
bike1.printStates(); // call the printStates() method of the bike1 object
System.out.print("Bike 2 starting values are -> ");
bike2.printStates(); // call the printStates() method of the bike2 object
System.out.print("Bike 3 starting values are -> ");
bike3.printStates(); // call the printStates() method of the bike3 object
bike1.changeCadence(50); // call the changeCadence() method of the bike1 object. send 50 as the value to be used
bike1.speedUp(10); // call the speedUp() method of the bike1 object. send 10 as the value to be used
bike1.changeGear(2); // call the changeGear() method of the bike1 object. send 2 as the value to be used
System.out.print("Bike 1 values are now -> ");
bike1.printStates(); // call the printStates() method of the bike1 object
bike2.changeCadence(60); // call the changeCadence() method of the bike2 object. send 60 as the value to be used
bike2.speedUp(12); // call the speedUp() method of the bike2 object. send 12 as the value to be used
bike2.changeGear(3); // call the changeGear() method of the bike2 object. send 3 as the value to be used
System.out.print("Bike 2 values are now -> ");
bike2.printStates(); // call the printStates() method of the bike2 object
bike2.changeCadence(40); // call the changeCadence() method of the bike2 object. send 40 as the value to be used
bike2.speedUp(10); // call the speedUp() method of the bike2 object. send 10 as the value to be used
bike2.changeGear(2); // call the changeGear() method of the bike2 object. send 2 as the value to be used
System.out.print("Bike 2 values are now -> ");
bike2.printStates(); // call the printStates() method of the bike2 object
bike3.changeCadence(90); // call the changeCadence() method of the bike3 object. send 90 as the value to be used
bike3.speedUp(5); // call the speedUp() method of the bike3 object. send 5 as the value to be used
System.out.print("Bike 3 values are now -> ");
bike3.printStates(); // call the printStates() method of the bike3 object
}
}
The output of this test prints the ending pedal cadence, speed, and gear for the two bicycles:
Bike 1 starting values are -> cadence:0 speed:0 gear:0
Bike 2 starting values are -> cadence:0 speed:0 gear:0
Bike 3 starting values are -> cadence:76 speed:28 gear:3
Bike 1 values are now -> cadence:50 speed:10 gear:2
Bike 2 values are now -> cadence:60 speed:12 gear:3
Bike 2 values are now -> cadence:40 speed:22 gear:2
Bike 3 values are now -> cadence:90 speed:33 gear:3
To view the above code as an actual BlueJ Project follow these steps:
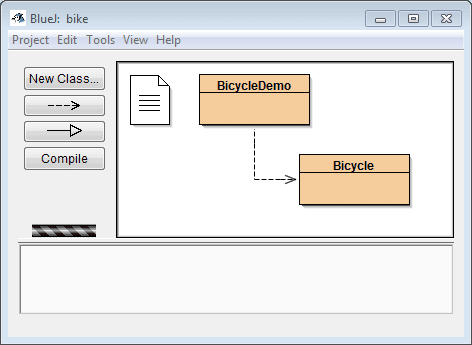
- Open BlueJ and create a new project named bike.
- Add a Class named Bicycle (be sure to capitalize the word Bicycle)
- Copy the Class Bicycle code from above and paste it into the Bicycle Class you created in BlueJ
- Compile the code for the Bicycle Class
- Create a new class named BicycleDemo
- Copy the Class BicycleDemo code from above and paste it into the BicycleDemo Class you created in BlueJ
- Compile the code for the BicycleDemo Class
- Right-click on the BicycleDemo Class and select void main(String[] args)
- Press the OK button.
- The output will be as shown in the image below:
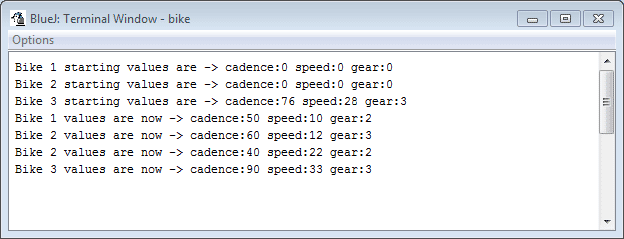
|