|
To complete this set of problems you must have gone through the following lessons:
- All lessons for Problem Sets 1, 2, 3 & 4
- Arrays (single)
- Arrays (double)
Problem 1 |
Create an array that has 4 columns and 3 rows. Store in each cell a letter of the alphabet. Have the computer print a table of the entered letters. Then have the computer print a table in which the rows and columns are switched as shown on the right. |
Output Example |
Enter a letter: A
Enter a letter: B
Enter a letter: C
Enter a letter: D
Enter a letter: E
Enter a letter: F
Enter a letter: G
Enter a letter: H
Enter a letter: I
Enter a letter: J
Enter a letter: K
Enter a letter: L
Switched...
|
Problem 2 |
Dr. Bonebreak wants you to program the computer to keep track of his busy schedule. (A) Write a program to allow a patient to choose the day and time he or she wants to see the doctor. There are five days and six time slots for each day. If the desired slot is empty, the patient enters his or her name. If it is full, the program then asks for another slot. (B) Add the steps needed to allow Dr. Bonebreak to display his schedule for any particular day. Have the program terminate when X is entered for the day. |
Output Example |
Please enter your name: Joe Schmoe
Please enter the day (M,T,W,R,F): T
Please enter the time slot (1-6): 2
Your name has been entered.
Please enter your name: Betty Boop
Please enter the day (M,T,W,R,F): T
Please enter the time slot (1-6): 2
That time slot is taken please choose another.
Please enter the day (M,T,W,R,F): T
Please enter the time slot (1-6): 3
Your name has been entered.
Please enter your name: Dr. Bonebreak
Welcome Dr. Bonebreak.You are now logged in.
Enter the day to see (Z to quit): T
Slot 1 Empty
Slot 2 Joe Schmoe
Slot 3 Betty Boop
Slot 4 Empty
Slot 5 Empty
Slot 6 Empty
Welcome Dr. Bonebreak.
Enter the day to see (Z to quit): Z
Good-bye Dr. Bonebreak.
Please enter your name: Joe Schmoe
Please enter the day (M,T,W,R,F): X
Program terminated |
Problem 3 |
Write a program that asks for 12 different numbers to be entered. The computer should randomly assign them to cells of a two dimensional array that has 4 rows and 3 columns. There must be one number assigned to each cell with no cells left empty. |
Output Example |
Enter number 1: 1
Enter number 2: 2
Enter number 3: 3
Enter number 4: 4
Enter number 5: 5
Enter number 6: 6
Enter number 7: 7
Enter number 8: 8
Enter number 9: 9
Enter number 10: 10
Enter number 11: 11
Enter number 12: 12
The assigned places for the numbers entered are:
12 |
9 |
1 |
2 |
5 |
3 |
11 |
7 |
10 |
8 |
4 |
6 |
|
Problem 4 |
The game Penny Pitch is common in amusement parks. Pennies are tossed onto a checkerboard on which numbers have been printed. By adding up the numbers in the squares on which the pennies fall, a score is accumulated. Write a program that simulates this game in which ten pennies are to be randomly pitched onto the board shown below. Do not allow the computer to select the same space more than once.
1 |
1 |
1 |
1 |
1 |
1 |
1 |
2 |
2 |
2 |
2 |
1 |
1 |
2 |
3 |
3 |
2 |
1 |
1 |
2 |
3 |
3 |
2 |
1 |
1 |
2 |
2 |
2 |
2 |
1 |
1 |
1 |
1 |
1 |
1 |
1 |
|
Output Example |
1 |
1 |
X |
1 |
1 |
1 |
1 |
2 |
2 |
2 |
2 |
1 |
1 |
X |
3 |
3 |
X |
1 |
X |
X |
3 |
X |
X |
1 |
1 |
2 |
2 |
X |
2 |
1 |
1 |
1 |
X |
X |
1 |
1 |
Your score: 17 |
Problem 5 |
Write a program that pits a human against a computer in a game of Tic-tac-toe. Use a String array with three rows and three columns. The human should be given the option of starting first or second. The program must prevent cheating of any kind. |
Output Example |
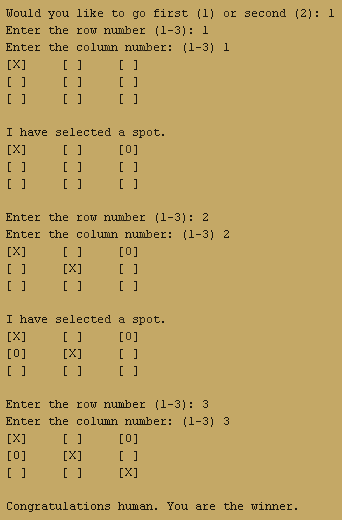 |
|
|
|