Assigning variables the data they will hold can take place in a number of ways. You can simply type a line of code that says something like: myName = "Jim";
But, what if you won't know the value of the variable until the program is running? For example, you may want to know the name of the user. So, during the run of the program a prompt appears on the screen asking the user to enter a name. After the user enters a name and presses the Enter key that is when the computer assigns the variable its data.
To make it possible for us to be able to assign variable values during the run of the program we will need to have our program first create an object that knows how to grab information entered by the user. (This is digging into something called OOP, Object Oriented Programming, that is used in the Programming 2 course. For now you can simply use the code shown below without understanding the details about why it works. You know, like when you drive a car but you don't know how the motor works.) First, before you can create a scanner object you will need to import the Java library that has the code for scanner objects. To import this library add the following line of code to the very top of your project. (NOTICE: This code already exists in your default code because you are using the stdclass.tmpl file that was modified for this class.) import java.util.*;
To create a Scanner object used to scan the Terminal Window for user input first create the Scanner object:. (NOTICE: This code already exists in your default code because you are using the stdclass.tmpl file that was modified for this class.) Scanner in = new Scanner(System.in);
Then in the method, declare the appropriate variable(s) that you will use for holder user input:
String name; double num1,num2,answer; int wholeNum;
Finally, in the method write the code for the user prompt followed by the line of code that assigns the variable the data coming from the user:
Text String input example:
System.out.print("Enter your name: "); name= in.next(); //used to input a String
Decimal double number input example:
System.out.print("Enter a decimal number: "); num1 = in.nextDouble(); //used to input a double
Integer int number input example:
System.out.print("Enter a whole number: "); wholeNum = in.nextInt(); //used to input a double
For each of the Programming 1 lessons you are strongly encouraged to view and play around with the sample code provided in the BlueJ Java Student Samples folder. Open BlueJ and choose Open Project from the Project menu. Navigate to your Programming 1 folder on the I: drive and open the BlueJ Java Student Samples folder. Open the project named first. 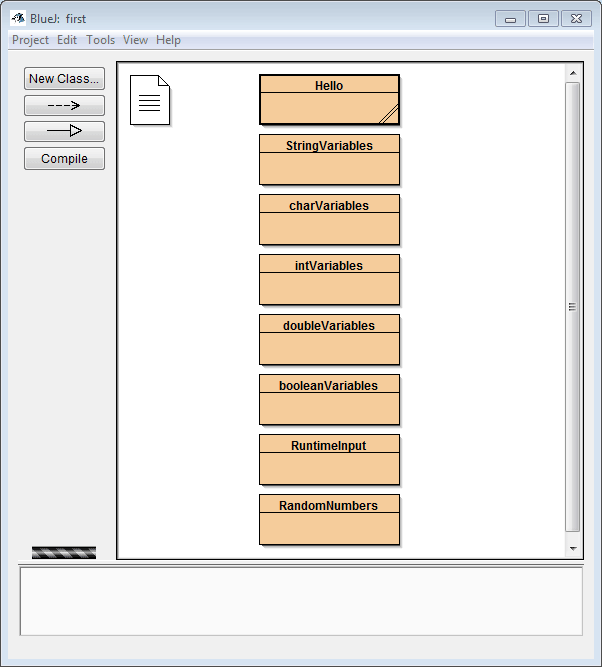
Lesson Example: Project first, Class RuntimeInput, method assignedInCode() - Right-click on the Class named RuntimeInput and select new RuntimeInput()
- Right-click on the red rectangle and choose the method named assignedInCode()
Code for method assignedInCode(): void assignedInCode() { String name; double num1,num2,answer; int wholeNum; System.out.print("Enter your name: "); name= in.next(); //used to input a String System.out.print("Enter a decimal number: "); num1 = in.nextDouble(); //used to input a double System.out.print("Enter another decimal number: "); num2 = in.nextDouble(); //used to input a double System.out.print("Enter a whole number: "); wholeNum = in.nextInt(); //used to input a double answer=num1/num2; answer=Math.round(answer*100.0)/100.0; //used to round to 2 decimal places System.out.println("Hey " + name + ", " + num1 + " / " + num2 + " = " + answer); System.out.println("The whole number entered is " + wholeNum); }
|