double variables are used to store decimal numbers. Most numbers used in a program are of this type.
Sample double variables:
double loan_rate = .09;
double length = 12.55;
double weight = 178.9;
Special Notice: when using decimal numbers in a calculation the results will usually have a large number of decimal places. The following code samples illustrate a method to format the output to an appropriate number of decimal places.
Format output to three decimal places: miles = Math.round(miles*1000.0)/1000.0;
Format output to two decimal places: miles = Math.round(miles*100.0)/100.0;
Format output to one decimal place: miles = Math.round(miles*10.0)/10.0;
For each of the Programming 1 lessons you are strongly encouraged to view and play around with the sample code provided in the BlueJ Java Student Samples folder.
Open BlueJ and choose Open Project from the Project menu. Navigate to your Programming 1 folder on the I: drive and open the BlueJ Java Student Samples folder. Open the project named first.
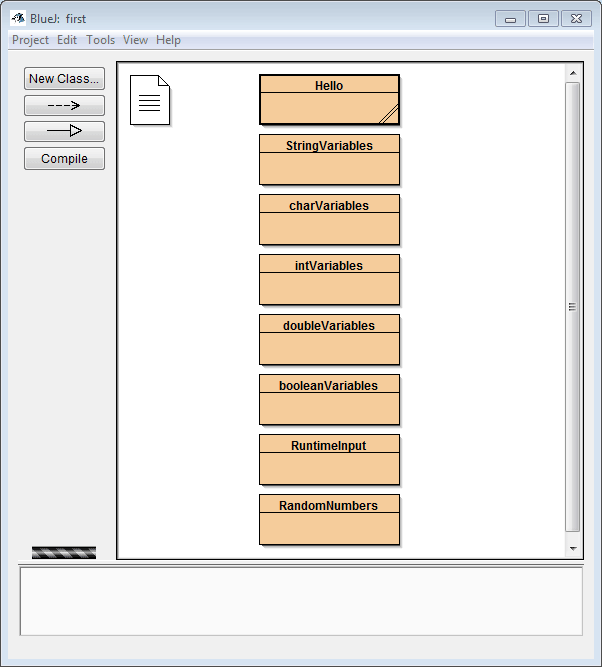
Lesson Example: Project first, Class doubleVariables, methods usingDouble and doubleDivision
- Right-click on the Class named doubleVariables and select new doubleVariables()
- Right-click on the red rectangle and choose the method named usingDouble()
- Right-click on the red rectangle and choose the method named doubleDivision()
Code for method usingDouble():
void usingDouble()
{
double x,y,z;
x=3.2;
y=8.51;
z=x+y;
System.out.println(x + " + " + y + " = " + z);
}
Code for doubleDivision():
void doubleDivision()
{
double x,y,z;
x=17;
y=3;
z=x/y;
System.out.println(x + "/" + y + " = " + z);
}