Manipulating Characters in a String
The String class has a number of methods for examining the contents of strings, finding characters or substrings within a string, changing case, and other tasks.
Getting Characters and Substrings by Index
You can get the character at a particular index within a string by invoking the charAt() accessor method. The index of the first character is 0, while the index of the last character is length()-1. For example, the following code gets the character at index 9 in a string:
String anotherPalindrome = "Niagara. O roar again!";
char aChar = anotherPalindrome.charAt(9);
Indices begin at 0, so the character at index 9 is 'O', as illustrated in the following figure:
If you want to get more than one consecutive character from a string, you can use the substring method. The substring method has two versions, as shown in the following table:
The substring Methods in the String Class |
Method |
Description |
String substring(int beginIndex, int endIndex) |
Returns a new string that is a substring of this string. The first integer argument specifies the index of the first character. The second integer argument is the index of the last character + 1. |
String substring(int beginIndex) |
Returns a new string that is a substring of this string. The integer argument specifies the index of the first character. Here, the returned substring extends to the end of the original string. |
The following code gets from the Niagara palindrome the substring that extends from index 11 up to, but not including, index 15, which is the word "roar":
String anotherPalindrome = "Niagara. O roar again!";
String roar = anotherPalindrome.substring(11, 15);
Other Methods for Manipulating Strings
Here are several other String methods for manipulating strings:
Other Methods in the String Class for Manipulating Strings |
Method |
Description |
String[] split(String regex)
String[] split(String regex, int limit) |
Searches for a match as specified by the string argument (which contains a regular expression) and splits this string into an array of strings accordingly. The optional integer argument specifies the maximum size of the returned array. |
CharSequence subSequence(int beginIndex, int endIndex) |
Returns a new character sequence constructed from beginIndex index up until endIndex - 1. |
String trim() |
Returns a copy of this string with leading and trailing white space removed. |
String toLowerCase()
String toUpperCase() |
Returns a copy of this string converted to lowercase or uppercase. If no conversions are necessary, these methods return the original string. |
Searching for Characters and Substrings in a String
Here are some other String methods for finding characters or substrings within a string. The String class provides accessor methods that return the position within the string of a specific character or substring: indexOf() and lastIndexOf(). The indexOf() methods search forward from the beginning of the string, and the lastIndexOf() methods search backward from the end of the string. If a character or substring is not found, indexOf() and lastIndexOf() return -1.
The String class also provides a search method, contains, that returns true if the string contains a particular character sequence. Use this method when you only need to know that the string contains a character sequence, but the precise location isn't important.
The following table describes the various string search methods.
The Search Methods in the String Class |
Method |
Description |
int indexOf(int ch)
int lastIndexOf(int ch) |
Returns the index of the first (last) occurrence of the specified character. |
int indexOf(int ch, int fromIndex)
int lastIndexOf(int ch, int fromIndex) |
Returns the index of the first (last) occurrence of the specified character, searching forward (backward) from the specified index. |
int indexOf(String str)
int lastIndexOf(String str) |
Returns the index of the first (last) occurrence of the specified substring. |
int indexOf(String str, int fromIndex)
int lastIndexOf(String str, int fromIndex) |
Returns the index of the first (last) occurrence of the specified substring, searching forward (backward) from the specified index. |
boolean contains(CharSequence s) |
Returns true if the string contains the specified character sequence. |
Note: CharSequence is an interface that is implemented by the String class. Therefore, you can use a string as an argument for the contains() method. |
Replacing Characters and Substrings into a String
The String class has very few methods for inserting characters or substrings into a string. In general, they are not needed: You can create a new string by concatenation of substrings you have removed from a string with the substring that you want to insert.
The String class does have four methods for replacing found characters or substrings, however. They are:
Methods in the String Class for Manipulating Strings |
Method |
Description |
String replace(char oldChar, char newChar) |
Returns a new string resulting from replacing all occurrences of oldChar in this string with newChar. |
String replace(CharSequence target, CharSequence replacement) |
Replaces each substring of this string that matches the literal target sequence with the specified literal replacement sequence. |
String replaceAll(String regex, String replacement |
Replaces each substring of this string that matches the given regular expression with the given replacement. |
String replaceFirst(String regex, String replacement) |
Replaces the first substring of this string that matches the given regular expression with the given replacement. |
Comparing Strings and Portions of Strings
The String class has a number of methods for comparing strings and portions of strings. The following table lists these methods.
Methods for Comparing Strings |
Method |
Description |
boolean endsWith(String suffix)
boolean startsWith(String prefix) |
Returns true if this string ends with or begins with the substring specified as an argument to the method. |
boolean startsWith(String prefix, int offset) |
Considers the string beginning at the index offset, and returns true if it begins with the substring specified as an argument. |
int compareTo(String anotherString) |
Compares two strings lexicographically. Returns an integer indicating whether this string is greater than (result is > 0), equal to (result is = 0), or less than (result is < 0) the argument. |
int compareToIgnoreCase(String str) |
Compares two strings lexicographically, ignoring differences in case. Returns an integer indicating whether this string is greater than (result is > 0), equal to (result is = 0), or less than (result is < 0) the argument. |
boolean equals(Object anObject) |
Returns true if and only if the argument is a String object that represents the same sequence of characters as this object. |
boolean equalsIgnoreCase(String anotherString) |
Returns true if and only if the argument is a String object that represents the same sequence of characters as this object, ignoring differences in case. |
boolean regionMatches(int toffset, String other, int ooffset, int len) |
Tests whether the specified region of this string matches the specified region of the String argument.
Region is of length len and begins at the index toffset for this string and ooffset for the other string. |
boolean regionMatches(boolean ignoreCase, int toffset, String other, int ooffset, int len) |
Tests whether the specified region of this string matches the specified region of the String argument.
Region is of length len and begins at the index toffset for this string and ooffset for the other string.
The boolean argument indicates whether case should be ignored; if true, case is ignored when comparing characters. |
boolean matches(String regex) |
Tests whether this string matches the specified regular expression. |
The following program, RegionMatchesDemo, uses the regionMatches method to search for a string within another string:
public class RegionMatchesDemo
{
public static void main(String[] args)
{
String searchMe = "Green Eggs and Ham";
String findMe = "Eggs";
int searchMeLength = searchMe.length();
int findMeLength = findMe.length();
boolean foundIt = false;
for (int i = 0; i <= (searchMeLength - findMeLength); i++)
{
if (searchMe.regionMatches(i, findMe, 0, findMeLength))
{
foundIt = true;
System.out.println(searchMe.substring(i, i + findMeLength));
break;
}
}
if (!foundIt) System.out.println("No match found.");
}
}
The output from this program is: Eggs.
The program steps through the string referred to by searchMe one character at a time. For each character, the program calls the regionMatches method to determine whether the substring beginning with the current character matches the string the program is looking for.
Unicode
Each keyboard character has a numeric equivalent. It is possible to find the number that represents a key by using (int). It is also possible to do the reverse by using (char)
The following code demonstrates the use of (int) and (char):
import java.util.*;
public class Unicode
{
Scanner in = new Scanner(System.in);
void unicode_to_char()
{
int num;
System.out.print("Enter a number from 0 to 255: ");
num = in.nextInt();
System.out.println(num + " represents the character '" + (char)(num) + "'.");
}
void char_to_unicode()
{
char inputItem;
String text;
System.out.print("Enter a single character: ");
text = in.next();
inputItem = text.charAt(0);
if(Character.isLetter(inputItem))
System.out.println("The letter '" + inputItem + "' is " + (int)(inputItem) + " in unicode.");
else if(Character.isDigit(inputItem))
System.out.println("The digit '" + inputItem + "' is " + (int)(inputItem) + " in unicode.");
else if(Character.isWhitespace(inputItem))
System.out.println("That character(Space, Tab, Enter) is " + (int)(inputItem) + " in unicode.");
else
System.out.println("The character '" + inputItem + "' is " + (int)(inputItem) + " in unicode.");
}
}
The follow chart shows the unicode values for many of the characters:
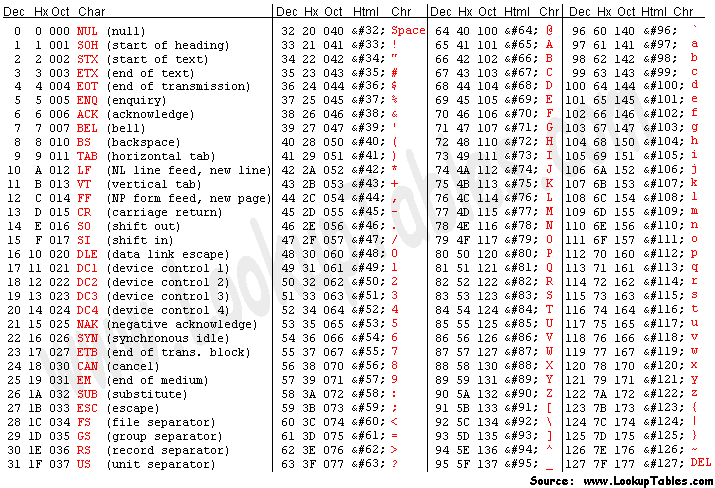
For each of the Programming 1 lessons you are strongly encouraged to view and play around with the sample code provided in the BlueJ Java Student Samples folder.
Open BlueJ and choose Open Project from the Project menu. Navigate to your Programming 1 folder on the I: drive and open the BlueJ Java Student Samples folder. Open the project named Strings.
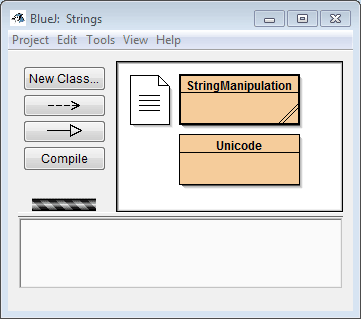
Lesson Example: Project Strings, Class StringManipulation, methods A_toLowerCase_String(), B_toUpperCase_String(), C_length_String(), D_trim_String(), E_startsWith_String(), F_endsWith_String(), G_compareTo_String(), H_compareToIgnoreCase_String(), I_equals_String(), J_equalsIgnoreCase_String(), K_concat_String(), L_replaceAll_String(), M_replace_String(), N_charAt_String(), O_indexOf_String(), P_anyIndexOf_String(), Q_lastIndexOf_String(), R_substring_Start_And_End_String() and S_substring_Start_To_End_String()
- Right-click on the Class named StringManipulations select new StringManipulations()
- Right-click on the red rectangle and choose the method named A_toLowerCase_String()
- Right-click on the red rectangle and choose the method named B_toUpperCase_String()
- Right-click on the red rectangle and choose the method named C_length_String()
- Right-click on the red rectangle and choose the method named D_trim_String()
- Right-click on the red rectangle and choose the method named E_startsWith_String()
- Right-click on the red rectangle and choose the method named F_endsWith_String()
- Right-click on the red rectangle and choose the method named G_compareTo_String()
- Right-click on the red rectangle and choose the method named H_compareToIgnoreCase_String()
- Right-click on the red rectangle and choose the method named I_equals_String()
- Right-click on the red rectangle and choose the method named J_equalsIgnoreCase_String()
- Right-click on the red rectangle and choose the method named K_concat_String()
- Right-click on the red rectangle and choose the method named L_replaceAll_String()
- Right-click on the red rectangle and choose the method named M_replace_String()
- Right-click on the red rectangle and choose the method named N_charAt_String()
- Right-click on the red rectangle and choose the method named O_indexOf_String()
- Right-click on the red rectangle and choose the method named P_anyIndexOf_String()
- Right-click on the red rectangle and choose the method named Q_lastIndexOf_String()
- Right-click on the red rectangle and choose the method named R_substring_Start_And_End_String()
- Right-click on the red rectangle and choose the method named S_substring_Start_To_End_String()
Code for method A_toLowerCase_String():
void A_toLowerCase_String()//converts String to all lowercase letters
{
String text;
System.out.print("Enter a word or phrase that uses upper case letters: ");
text = in.nextLine();
System.out.println("In lower case that looks like: " + text.toLowerCase());
}
Code for method B_toUpperCase_String():
void B_toUpperCase_String()//converts String to all uppercase letters
{
String text;
System.out.print("Enter a word or phrase that uses lower case letters: ");
text = in.nextLine();
System.out.println("In upper case that looks like: " + text.toUpperCase());
}
Code for method C_length_String():
void C_length_String()//finds the length of a String
{
String text;
int textLength;
System.out.print("Enter a word or phrase: ");
text = in.nextLine();
textLength = text.length();
System.out.println("The length of " + text + " is " + textLength);
}
Code for method D_trim_String():
void D_trim_String()//removes spaces before and after a String (but not inside the String)
{
String text;
System.out.print("Enter a word or phrase that has spaces before and/or after: ");
text = in.nextLine();
System.out.println("The text with spaces before and after removed looks like: " + text.trim());
}
Code for method E_startsWith_String():
void E_startsWith_String()//compares the start of a String with another String to see if they match
{
String text;
System.out.print("Enter the word 'pizza': ");
text = in.next();in.nextLine();
if(text.startsWith("piz"))
{
System.out.println("I think you wrote pizza because the first three letters are correct ");
System.out.println("but you might have entered pizzaz or some other word that starts with 'piz'.");
}
else
System.out.println("The word you entered does not start with piz.");
}
Code for method F_endsWith_String():
void F_endsWith_String()//compares the end of a String with another String to see if they match
{
String text;
System.out.print("Enter the word 'helicopter': ");
text = in.next();in.nextLine();
if(text.endsWith("copter"))
{
System.out.println("I think you wrote helicopter because the last six letters are correct ");
System.out.println("but you might have entered 'gyrocopter' or some other word that ends with 'copter'.");
}
else
System.out.println("The word you entered does not end with copter.");
}
Code for method G_compareTo_String():
void G_compareTo_String()//compares two Strings alphabetically - is case sensitive
{
String firstWord,secondWord;
System.out.print("Enter a word: ");
firstWord = in.next();in.nextLine();
System.out.print("Enter another word: ");
secondWord = in.next();in.nextLine();
if(firstWord.compareTo(secondWord)< 0)
System.out.println(firstWord + " comes before " + secondWord + " alphabetically.");
else if (firstWord.compareTo(secondWord)> 0)
System.out.println(firstWord + " comes after " + secondWord + " alphabetically.");
else if(firstWord.compareTo(secondWord)== 0)// also works: if(firstWord.equals(secondWord))
System.out.println(firstWord + " and " + secondWord + " are the same.");
}
Code for method H_compareToIgnoreCase_String():
void H_compareToIgnoreCase_String()//compares two Strings alphabetically - is not case sensitive
{
String firstWord,secondWord;
System.out.print("Enter a word using lowercase letters: ");
firstWord = in.next();in.nextLine();
System.out.print("Enter the same word using uppercase letters: ");
secondWord = in.next();in.nextLine();
if(firstWord.compareToIgnoreCase(secondWord)== 0)
System.out.println(firstWord + " and " + secondWord + " are the same.");
else
System.out.println(firstWord + " and " + secondWord + " are not the same.");
}
Code for method I_equals_String():
void I_equals_String()//compares two Strings to see if they are spelled indentically - is case sensitive
{
String firstWord,secondWord;
System.out.print("Enter a word: ");
firstWord = in.next();in.nextLine();
System.out.print("Enter another word: ");
secondWord = in.next();in.nextLine();
if(firstWord.equals(secondWord))
System.out.println(firstWord + " and " + secondWord + " are identical.");
else
System.out.println(firstWord + " and " + secondWord + " are not identical.");
}
Code for method J_equalsIgnoreCase_String():
void J_equalsIgnoreCase_String()//compares two Strings to see if they are spelled the same - is not case sensitive
{
String firstWord,secondWord;
System.out.print("Enter a word using lowercase letters: ");
firstWord =in.next();in.nextLine();
System.out.print("Enter the same word using uppercase letters: ");
secondWord = in.next();in.nextLine();
if(firstWord.equalsIgnoreCase(secondWord))
System.out.println(firstWord + " and " + secondWord + " are spelled the same.");
else
System.out.println(firstWord + " and " + secondWord + " are not spelled the same.");
}
Code for method K_concat_String():
void K_concat_String()//attaches Srtings together
{
String firstWord,secondWord,bothWords;
System.out.print("Enter a word: ");
firstWord = in.next();in.nextLine();
System.out.print("Enter another word: ");
secondWord = in.next();in.nextLine();
bothWords = firstWord.concat(secondWord);
System.out.println("The two words put together are: " + bothWords);
}
Code for method L_replaceAll_String():
void L_replaceAll_String()//replaces all occurrences of a String within another String, even individual letters
{
String text,wrd,rplc;
System.out.print("Enter a phrase: ");
text = in.nextLine();
System.out.print("Enter a word to be replaced in the phrase: ");
wrd =in.next();in.nextLine();
System.out.print("Enter the replacement word: ");
rplc = in.next();in.nextLine();
System.out.println("The new phrase is: " + text.replaceAll(wrd,rplc));
}
Code for method M_replace_String():
void M_replace_String()//replaces only individual letters in a String
{
String text;
char letterToReplace; //notice the use of char because you can only replace a char not a String
char replacementLetter; //notice the use of char because you can only replace a char not a String
String letter1;
String letter2;
System.out.print("Enter a word or phrase: ");
text = in.nextLine();
System.out.print("Enter a letter to replace in the text entered: ");
letter1 = in.next();in.nextLine();
System.out.print("Enter a letter to use as a replacement: ");
letter2 = in.next();in.nextLine();
letterToReplace=letter1.charAt(0); //notice conversion of a String to a char
replacementLetter=letter2.charAt(0); //notice conversion of a String to a char
System.out.println("Replacing " + letterToReplace + " with " + replacementLetter + " gives: " + text.replace(letterToReplace,replacementLetter));
}
Code for method N_charAt_String():
void N_charAt_String()//finds the character in a String at a given position
{
String text="";
int position=0;
char found_char;
System.out.print("Enter a word or phrase: ");
text = in.nextLine();
System.out.print("Enter a number within the length of the entered text: ");
position = in.nextInt();in.nextLine();
if (position >=0 & position <= text.length())
{
found_char=text.charAt(position);
System.out.println("Starting with the first character as 0, the character in that position is: " + found_char);
}
else
System.out.println("That number is out of range.");
}
Code for method O_indexOf_String():
void O_indexOf_String()//finds the numeric position of the first occurrence of String within a String
{
String text;
String letter;
int position;
System.out.print("Enter a word or phrase: "); // try cheeseburger
text = in.nextLine();
System.out.print("Enter a letter or group of letters found in the word or phrase you entered: "); // enter e
letter = in.next();in.nextLine();
position = text.indexOf(letter);
System.out.println("The first occurrence of " + letter + " is found at position " + position);
}
Code for method P_anyIndexOf_String():
void P_anyIndexOf_String()//finds the numeric position of the first occurrence of a String within a String from a given position
{
String text;
int position,start_pos;
System.out.print("Enter a word or phrase that uses the letter 'e' multiple times: "); // try cheeseburger
text = in.nextLine();
System.out.print("Enter a position from where searching for an 'e' will begin: "); // enter e
start_pos = in.nextInt();in.nextLine();
position = text.indexOf("e",start_pos);
System.out.println("The first occurrence of the letter 'e' after the starting position entered is found at " + position);
}
Code for method Q_lastIndexOf_String():
void Q_lastIndexOf_String()//finds the numeric position last occurrence of a String within a String
{
String text;
String letter;
int position;
System.out.print("Enter a word or phrase: "); // try cheeseburger
text = in.nextLine();
System.out.print("Enter a letter or group of letters found in the word or phrase you entered: "); // enter e
letter = in.next();in.nextLine();
position = text.lastIndexOf(letter);
System.out.println("The last occurrence of " + letter + " is found at position " + position);
}
Code for method R_substring_Start_And_End_String():
void R_substring_Start_And_End_String()//returns a part of a String starting from a given position and ending at another given position
{
String text;
String theSubstring;
int positionToStart;
int positionToEnd;
System.out.print("Enter a word: ");
text = in.next();in.nextLine();
System.out.print("Enter a number to start the substring: ");
positionToStart = in.nextInt();in.nextLine();
System.out.print("Enter a number to end the substring: ");
positionToEnd = in.nextInt();in.nextLine();
theSubstring = text.substring(positionToStart,positionToEnd);
System.out.println("Starting at position " + positionToStart + " and ending one letter BEFORE " + positionToEnd + " characters, results in: " + theSubstring);
}
Code for method S_substring_Start_To_End_String():
void S_substring_Start_To_End_String()//returns a part of a String starting from a given position to the end of the String
{
String text;
String theSubstring;
int positionToStart;
System.out.print("Enter a word: ");
text = in.next();in.nextLine();
System.out.print("Enter a number to start the substring: ");
positionToStart = in.nextInt();in.nextLine();
theSubstring = text.substring(positionToStart);
System.out.println("Starting at position " + positionToStart + " and going to the ending results in: " + theSubstring);
}
|