The If statement allows the computer to evaluate a condition and then act on the results. In other words, it allows the computer to make decisions about what action to take next. For example, suppose a game playing user has the score of 100 points and that is the amount necessary to win. The computer can look at the score and compare it to the amount needed to win and see that they are equal. With that knowledge the computer can take the action of declaring the user a winner.
General form of If statement:
if (condition is true)
execute this code;
What if the condition is not true? In the sample above nothing would happen. We did not tell the computer what action to take if the condition is false. To remedy this problem view the following code sample.
General form of If with else statement:
if (condition is true)
execute this code;
else
execute this code;
The above code supplies the computer with the action that is to be taken if the condition is true. The code following the else line is the code executed if the condition is false.
What if there are more than two possible outcomes? For example you might say if it is raining you will bring an umbrella, if it is snowing you will wear boots and if the weather is nice you will wear tennis shoes. Now there are three possible outcomes. To remedy this problem view the following code sample.
General form of If with else if and else statement:
if (condition is true)
execute this code;
else if (another condition is true)
execute this code;
else
execute this code;
The above code handles three possible outcomes. Often there are many more than three outcomes. The pattern of how many if, else if and else lines to write looks like this:
For four possible outcomes:
if()
else if()
else if()
else
For five possible outcomes:
if()
else if()
else if()
else if()
else
etc...
For each of the Programming 1 lessons you are strongly encouraged to view and play around with the sample code provided in the BlueJ Java Student Samples folder.
Open BlueJ and choose Open Project from the Project menu. Navigate to your Programming 1 folder on the I: drive and open the BlueJ Java Student Samples folder. Open the project named If.
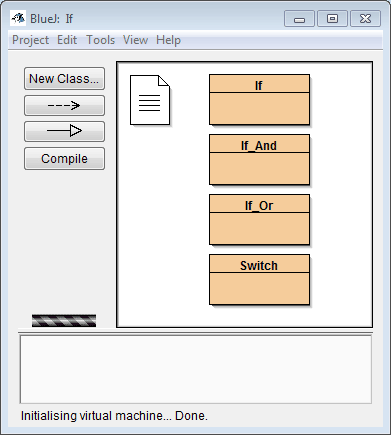
Lesson Example: Project If, Class If, methods simpleIf(), ifWithElse(), ifWithElseIf() and usingBlocksWithIf()
- Right-click on the Class named If and select new If()
- Right-click on the red rectangle and choose the method named simpleIf()
- Right-click on the red rectangle and choose the method named ifWithElse()
- Right-click on the red rectangle and choose the method named ifWithElseIf()
- Right-click on the red rectangle and choose the method named usingBlocksWithIf()
Code for method simpleIf():
void simpleIf()
{
int num1,num2;
System.out.print("Enter a number: ");
num1 = in.nextInt();
System.out.print("Enter another number: ");
num2 = in.nextInt();
if (num1>num2)
System.out.println(num1 + " is bigger than " + num2);
}
Code for method ifWithElse():
void ifWithElse()
{
int num1,num2;
System.out.print("Enter a number: ");
num1 = in.nextInt();
System.out.print("Enter another number: ");
num2 = in.nextInt();
if (num1>num2)
System.out.println(num1 + " is bigger than " + num2);
else
System.out.println(num1 + " is smaller than " + num2 + " or they are the same.");
}
Code for method ifWithElseIf():
void ifWithElseIf()
{
int num1,num2;
System.out.print("Enter a number: ");
num1 = in.nextInt();
System.out.print("Enter another number: ");
num2 = in.nextInt();
if (num1>num2)
System.out.println(num1 + " is bigger than " + num2);
else if (num1<num2)
System.out.println(num1 + " is smaller than " + num2);
else
System.out.println(num1 + " is the same as " + num2);
}
Code for method usingBlocksWithIf():
void usingBlocksWithIf()
{
int num1,num2;
System.out.print("Enter a number: ");
num1 = in.nextInt();
System.out.print("Enter another number: ");
num2 = in.nextInt();
if (num1>num2)
{
System.out.println(num1 + " is bigger than " + num2);
System.out.println(num1 + " is the greatest number!");
}
else if (num1<num2)
System.out.println(num1 + " is smaller than " + num2);
else
{
System.out.println(num1 + " is the same as " + num2);
System.out.println(num1 + " has met it's match.");
}
}