Random Number Generation It is often very useful to be able to generate numbers randomly. For example, a game may ask a user to guess a number from 1 to 100. If the game had the same number every time or the pattern of numbers could be determined then the game would be of no use.
The general code to generate a random number from low to high follows this form: int variable of your choice = (int)(Math.random() * highest possible choice - lowest possible choice + 1) + lowest possible choice; To generate random numbers use the following code: (assumes the int variable rndmNum has been declared) - Generate a number from 1 to 10: rndmNum = (int)(Math.random() * 10) + 1;
- Generate a number from 50 to 75: rndmNum = (int)(Math.random() * 26) + 50;
For each of the Programming 1 lessons you are strongly encouraged to view and play around with the sample code provided in the BlueJ Java Student Samples folder. Open BlueJ and choose Open Project from the Project menu. Navigate to your Programming 1 folder on the I: drive and open the BlueJ Java Student Samples folder. Open the project named first. 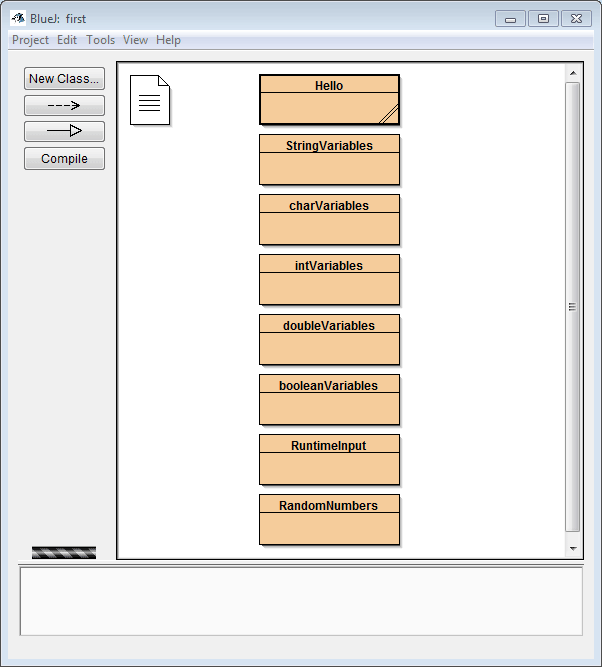
Lesson Example: Project first, Class RandomNumbers, method generateRandomNumber() - Right-click on the Class named RandomNumbers and select new RandomNumbers ()
- Right-click on the red rectangle and choose the method named generateRandomNumber()
Code for method generateRandomNumber(): void generateRandomNumber() { int rndmNum; rndmNum = (int)(Math.random() * 10) + 1;//inside value is high-low+1 System.out.println("The number chosen from 1 to 10 is: " + rndmNum); rndmNum = (int)(Math.random() * 6) + 15; System.out.println("The number chosen from 15 to 20 is: " + rndmNum); }
|